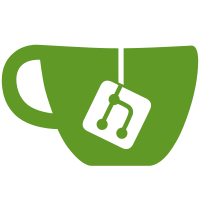
import sys import yaml with open(sys.argv[1]) as fp: data = fp.read() if not data.find("---") == 0: # no head print("NO YAML HEAD FOUND") sys.exit(-1) data = data[3:] head_end = data.find("---") head = data[0:head_end] data = data[head_end+3:] metadata = yaml.safe_load(head) cats = metadata.pop('categories', None) if cats != None: if type(cats) == list: tags = cats elif type(cats) == str: tags = cats.split() tags = list(map(lambda t: t.lower(), tags)) metadata["tags"] = ", ".join(tags) new_data = f"---\n{yaml.dump(metadata, default_flow_style=False)}---{data}" # write it print(f"coverted: categories to tags: {tags} - {sys.argv[1]}") with open(sys.argv[1], "w") as fp: fp.write(new_data) sys.exit(0) if not metadata.get("tags", None): metadata["tags"] = "untagged" new_data = f"---\n{yaml.dump(metadata, default_flow_style=False)}---{data}" print(f"untagged: {sys.argv[1]}") # write it with open(sys.argv[1], "w") as fp: fp.write(new_data) sys.exit(0) print("No changes needed")
38 lines
1.4 KiB
Markdown
38 lines
1.4 KiB
Markdown
---
|
|
comments: true
|
|
date: 2013-06-23 19:40
|
|
layout: post
|
|
tags: rtems, gsoc, gdb
|
|
title: Debugging RTEMS with GDB
|
|
---
|
|
|
|
RTEMS is difficult to debug, since the default GDB behaviour follows a language based approch and developer will have to debug the application+RTEMS stack as a whole. We are in process of developing a new set of extenstions for GDB to play nice with RTEMS. The intial code is available in this [github repository](https://github.com/dbalan/rtems-gdb).
|
|
|
|
To use the extenstion,
|
|
- Clone the repository
|
|
{% codeblock lang:bash %}
|
|
git clone git@github.com:dbalan/rtems-gdb.git
|
|
{% endcodeblock %}
|
|
- Assuming you have a working [RTEMS toolchain](/blog/2013/05/28/getting-started-with-rtems-on-archlinux/), spin up the GDB and source the code.
|
|
{% codeblock lang:bash %}
|
|
$ sparc-rtems4.11-gdb
|
|
|
|
GNU gdb (GDB) 7.5.1
|
|
Copyright (C) 2012 Free Software Foundation, Inc.
|
|
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
|
|
This is free software: you are free to change and redistribute it.
|
|
There is NO WARRANTY, to the extent permitted by law. Type "show copying"
|
|
and "show warranty" for details.
|
|
This GDB was configured as "--host=x86_64-linux-gnu --target=sparc-rtems4.11".
|
|
For bug reporting instructions, please see:
|
|
<http://www.gnu.org/software/gdb/bugs/>.
|
|
(gdb) source path/to/clone/__init__.py
|
|
RTEMS GDB Support loaded
|
|
(gdb)
|
|
{% endcodeblock %}
|
|
|
|
Here is a sneak peak of what will be capable:
|
|
{% gist 5428535 %}
|
|
{% gist 5488653 %}
|
|
|