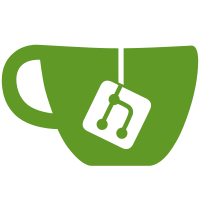
import sys import yaml with open(sys.argv[1]) as fp: data = fp.read() if not data.find("---") == 0: # no head print("NO YAML HEAD FOUND") sys.exit(-1) data = data[3:] head_end = data.find("---") head = data[0:head_end] data = data[head_end+3:] metadata = yaml.safe_load(head) cats = metadata.pop('categories', None) if cats != None: if type(cats) == list: tags = cats elif type(cats) == str: tags = cats.split() tags = list(map(lambda t: t.lower(), tags)) metadata["tags"] = ", ".join(tags) new_data = f"---\n{yaml.dump(metadata, default_flow_style=False)}---{data}" # write it print(f"coverted: categories to tags: {tags} - {sys.argv[1]}") with open(sys.argv[1], "w") as fp: fp.write(new_data) sys.exit(0) if not metadata.get("tags", None): metadata["tags"] = "untagged" new_data = f"---\n{yaml.dump(metadata, default_flow_style=False)}---{data}" print(f"untagged: {sys.argv[1]}") # write it with open(sys.argv[1], "w") as fp: fp.write(new_data) sys.exit(0) print("No changes needed")
38 lines
1.8 KiB
Markdown
38 lines
1.8 KiB
Markdown
---
|
|
author: dhananjayishere
|
|
comments: true
|
|
date: 2012-01-09 14:30:00
|
|
layout: post
|
|
slug: arrow-keys-input-in-python
|
|
tags: programing, python
|
|
title: Arrow Keys input in python.
|
|
wordpress_id: 93005403
|
|
---
|
|
|
|
I had an assignment to write an applicaion to control a toy helicopter. It should accept the inputs from the arrow keys and then generate a serial signal. The serial port is connected to the interfacing circutary.
|
|
|
|
The major problem I faced was how to take arrow keys as input? Using the technical jargon - implement a non-bufferd input. A code to do it in console can be found [here](http://code.activestate.com/recipes/134892-getch-like-unbuffered-character-reading-from-stdin/). But its dirty and is implemented in a complex way that usage is little bit diffcult. At console level the code becomes more os-specific, as you can see from the above code. It has diffrent defenitions to implement the feature in each os.
|
|
|
|
The easy way to do this is using any windowing tool kits around, they all have a key logging abstraction implemented. Like this [code](http://stackoverflow.com/a/4205490). it uses the tkinter toolkit to read input. The way I suggest is using pygame, because it is designed to this stuff. (Which game doesnt have a single use arrow key used?)
|
|
|
|
You can get the keys from
|
|
|
|
{% codeblock lang:python %}
|
|
pressed_keys = pygame.key.get_pressed()
|
|
{% endcodeblock %}
|
|
and the key name as
|
|
|
|
{% codeblock lang:python %}
|
|
for key_constant in pressed_keys:
|
|
key_name = pygame.key.name(key_constant)
|
|
{% endcodeblock %}
|
|
|
|
Then its just a matter of comparing them with the key name,( of arrow keys in our case).
|
|
|
|
{% codeblock lang:python %}
|
|
if key_constant == 'up':
|
|
port.write(_up_data)
|
|
{% endcodeblock %}
|
|
|
|
The complete code is available in [github](https://github.com/dhananjaynav/Scripts/blob/master/castalia/helicontrol.py)
|